diff options
-rw-r--r-- | Cargo.toml | 4 | ||||
-rw-r--r-- | README.md | 12 | ||||
-rw-r--r-- | assets/shaders/outline_post_process.wgsl | 72 | ||||
-rw-r--r-- | src/components.rs | 17 | ||||
-rw-r--r-- | src/lib.rs | 7 |
5 files changed, 63 insertions, 49 deletions
@@ -1,10 +1,10 @@ [package] name = "bevy_outline_post_process" -version = "0.4.0" +version = "0.5.0" edition = "2021" description = "An adaptive outline post-processing effect for the Bevy game engine." license = "0BSD OR MIT OR Apache-2.0" -repository = "https://git.exvacuum.dev/bevy_outline_post_process" +repository = "https://git.soaos.dev/bevy_outline_post_process" [dependencies.bevy] version = "0.15" @@ -7,20 +7,18 @@ A plugin for the [Bevy](https://bevyengine.org) engine which adds an outline post-processing effect. Optionally supports adaptive outlining, so darker areas are outlined in white rather than black, based on luminance. -Note: This is a full-screen post process effect and cannot be enabled/disabled for specific objects. - ## Screenshots 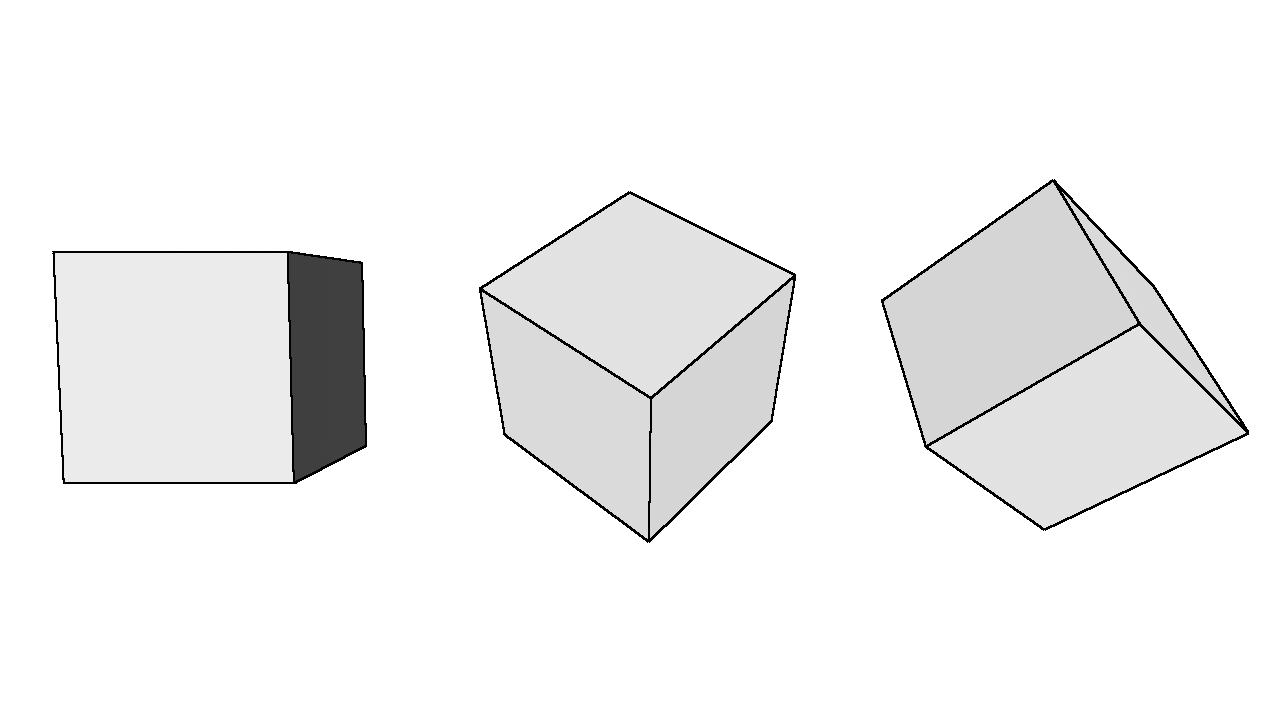 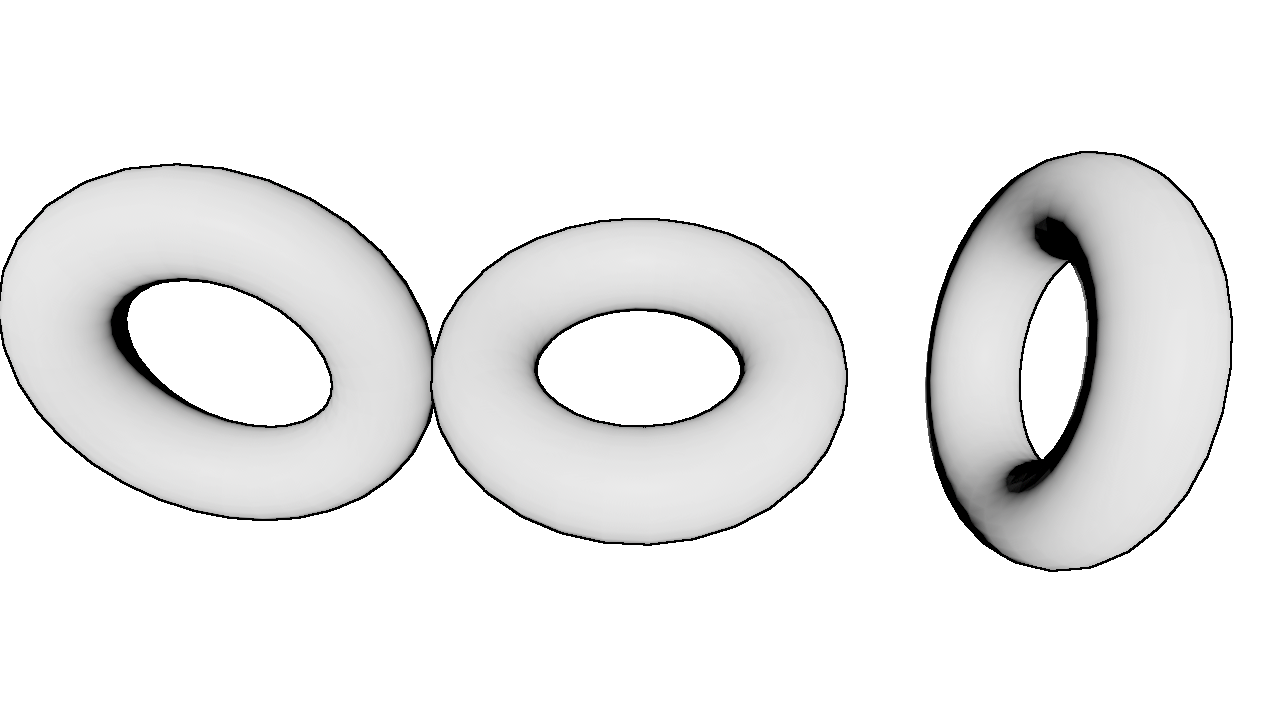 Configuration Used: ```rs -bevy_outline_post_process::components::OutlinePostProcessSettings::new(2.0, 0.0, false, 0.0); +bevy_outline_post_process::components::OutlinePostProcessSettings::new(2.0, LinearRgba::BLACK, 0.01, 0.01, 1.0); ``` ## Compatibility | Crate Version | Bevy Version | |--- |--- | -| 0.4 | 0.15 | +| 0.4-0.5 | 0.15 | | 0.3 | 0.14 | | 0.1-0.2 | 0.13 | @@ -29,13 +27,13 @@ bevy_outline_post_process::components::OutlinePostProcessSettings::new(2.0, 0.0, ### crates.io ```toml [dependencies] -bevy_outline_post_process = "0.4" +bevy_outline_post_process = "0.5" ``` ### Using git URL in Cargo.toml ```toml [dependencies.bevy_outline_post_process] -git = "https://git.exvacuum.dev/bevy_outline_post_process" +git = "https://git.soaos.dev/bevy_outline_post_process" ``` ## Usage @@ -59,7 +57,7 @@ When spawning a camera: ```rs commands.spawn(( // Camera3d... - bevy_outline_post_process::components::OutlinePostProcessSettings::new(2.0, 0.0, false, 0.0); + bevy_outline_post_process::components::OutlinePostProcessSettings::default(); )); ``` diff --git a/assets/shaders/outline_post_process.wgsl b/assets/shaders/outline_post_process.wgsl index e5263a1..8f05e01 100644 --- a/assets/shaders/outline_post_process.wgsl +++ b/assets/shaders/outline_post_process.wgsl @@ -1,15 +1,11 @@ #import bevy_core_pipeline::fullscreen_vertex_shader::FullscreenVertexOutput; -#import bevy_pbr::{ rgb9e5 }; -#import bevy_pbr::view_transformations::{ - perspective_camera_near, -}; -#import bevy_pbr::mesh_view_bindings as view_bindings; struct OutlinePostProcessSettings { weight: f32, + color: vec4<f32>, normal_threshold: f32, depth_threshold: f32, - adaptive: u32, + adaptive_threshold: f32, camera_near: f32, } @@ -30,58 +26,70 @@ fn fragment( let outline_width = settings.weight / vec2f(textureDimensions(screen_texture)); let uv_top = vec2f(in.uv.x, in.uv.y - outline_width.y); + let uv_bottom = vec2f(in.uv.x, in.uv.y + outline_width.y); let uv_right = vec2f(in.uv.x + outline_width.x, in.uv.y); + let uv_left = vec2f(in.uv.x - outline_width.x, in.uv.y); let uv_top_right = vec2f(in.uv.x + outline_width.x, in.uv.y - outline_width.y); // NORMAL FACTOR {{{ - let normal = textureSample(normal_texture, normal_sampler, in.uv); - let normal_top = textureSample(normal_texture, normal_sampler, uv_top); - let normal_right = textureSample(normal_texture, normal_sampler, uv_right); - let normal_top_right = textureSample(normal_texture, normal_sampler, uv_top_right); + let normal = textureSample(normal_texture, normal_sampler, in.uv).xyz; + let normal_top = textureSample(normal_texture, normal_sampler, uv_top).xyz; + let normal_right = textureSample(normal_texture, normal_sampler, uv_right).xyz; + let normal_top_right = textureSample(normal_texture, normal_sampler, uv_top_right).xyz; let normal_delta_top = abs(normal - normal_top); let normal_delta_right = abs(normal - normal_right); let normal_delta_top_right = abs(normal - normal_top_right); - let normal_delta_max = max(normal_delta_top, max(normal_delta_right, normal_delta_top_right)); - let normal_delta_raw = max(normal_delta_max.x, max(normal_delta_max.y, normal_delta_max.z)); + let normal_top_sum = max(normal_delta_top.x, max(normal_delta_top.y, normal_delta_top.z)); + let normal_right_sum = max(normal_delta_right.x, max(normal_delta_right.y, normal_delta_right.z)); + let normal_top_right_sum = max(normal_delta_top_right.x, max(normal_delta_top_right.y, normal_delta_top_right.z)); - let show_outline_normal = f32(normal_delta_raw > settings.normal_threshold); - let normal_outline = vec4f(show_outline_normal, show_outline_normal, show_outline_normal, 0.0); + var normal_difference = step(settings.normal_threshold, max(normal_top_sum, max(normal_right_sum, normal_top_right_sum))); + let normal_outline = normal_difference; // }}} - let frag_width = settings.weight / vec2f(textureDimensions(screen_texture)); - let depth_uv_top_right = vec2f(in.uv.x + frag_width.x, in.uv.y - frag_width.y); - let depth_uv_top_left = vec2f(in.uv.x - frag_width.x, in.uv.y - frag_width.y); - let depth_uv_bottom_right = vec2f(in.uv.x + frag_width.x, in.uv.y + frag_width.y); - let depth_uv_bottom_left = vec2f(in.uv.x - frag_width.x, in.uv.y + frag_width.y); // DEPTH FACTOR {{{ let depth_color = textureSample(depth_texture, depth_sampler, in.uv); + let depth_color_top = textureSample(depth_texture, depth_sampler, uv_top); + let depth_color_bottom = textureSample(depth_texture, depth_sampler, uv_bottom); + let depth_color_right = textureSample(depth_texture, depth_sampler, uv_right); + let depth_color_left = textureSample(depth_texture, depth_sampler, uv_left); + let depth = linearize_depth(depth_color); - let depth_top_right = linearize_depth(textureSample(depth_texture, depth_sampler, depth_uv_top_right)); - let depth_top_left = linearize_depth(textureSample(depth_texture, depth_sampler, depth_uv_top_left)); - let depth_bottom_right = linearize_depth(textureSample(depth_texture, depth_sampler, depth_uv_bottom_right)); - let depth_bottom_left = linearize_depth(textureSample(depth_texture, depth_sampler, depth_uv_bottom_left)); + let depth_top = linearize_depth(depth_color_top); + let depth_bottom = linearize_depth(depth_color_bottom); + let depth_right = linearize_depth(depth_color_right); + let depth_left = linearize_depth(depth_color_left); var depth_delta = 0.0; - depth_delta = depth_delta + depth - depth_top_right; - depth_delta = depth_delta + depth - depth_top_left; - depth_delta = depth_delta + depth - depth_bottom_right; - depth_delta = depth_delta + depth - depth_bottom_left; + depth_delta = depth_delta + depth - depth_top; + depth_delta = depth_delta + depth - depth_bottom; + depth_delta = depth_delta + depth - depth_right; + depth_delta = depth_delta + depth - depth_left; - var depth_outline = vec4(step(settings.depth_threshold, depth_delta)); + var depth_outline = step(settings.depth_threshold, depth_delta); if depth_color == 0.0 { - depth_outline = vec4(0.0); + depth_outline = 0.0; } // }}} - var outline = (depth_outline + normal_outline); - if settings.adaptive != 0 && luma < 0.5 { + var outline = saturate(depth_outline + normal_outline); + if (1.0 - luma) > settings.adaptive_threshold { outline = outline * -1; } - return screen_color - outline ; + if (outline == 1.0) { + return settings.color; + } + if (outline == -1.0) { + return vec4(vec3(1.0) - settings.color.xyz, 1.0); + } + return screen_color; } fn linearize_depth(depth: f32) -> f32 { + if depth == 0.0 { + return 0.0; + } return settings.camera_near / depth; } diff --git a/src/components.rs b/src/components.rs index bdce6c9..05a4594 100644 --- a/src/components.rs +++ b/src/components.rs @@ -11,14 +11,16 @@ use bevy::{ pub struct OutlinePostProcessSettings { /// Weight of outlines in pixels. weight: f32, + /// Color of outlines. + color: LinearRgba, /// A threshold for normal differences, values below this threshold will not become outlines. /// Higher values will result in more outlines which may look better on smooth surfaces. normal_threshold: f32, /// A threshold for depth differences (in units), values below this threshold will not become outlines. /// Higher values will result in more outlines which may look better on smooth surfaces. depth_threshold: f32, - /// Whether to use adaptive outlines. White outlines will be drawn around darker objects, while black ones will be drawn around lighter ones. - adaptive: u32, + /// Luma threshold to invert outline color. A value of `1.0` means this feature is disabled. + adaptive_threshold: f32, /// Near plane depth of camera, used for linearization of depth buffer values pub(crate) camera_near: f32, } @@ -27,15 +29,17 @@ impl OutlinePostProcessSettings { /// Create a new instance with the given settings pub fn new( weight: f32, + color: LinearRgba, normal_threshold: f32, depth_threshold: f32, - adaptive: bool, + adaptive_threshold: f32, ) -> Self { Self { weight, + color, normal_threshold, - adaptive: adaptive as u32, depth_threshold, + adaptive_threshold, camera_near: 0.0, } } @@ -45,9 +49,10 @@ impl Default for OutlinePostProcessSettings { fn default() -> Self { Self { weight: 1.0, - normal_threshold: 0.0, - adaptive: 0, + color: LinearRgba::BLACK, + normal_threshold: 0.01, depth_threshold: 0.05, + adaptive_threshold: 1.0, camera_near: 0.0, } } @@ -9,7 +9,9 @@ use bevy::{ core_pipeline::core_3d::graph::{Core3d, Node3d}, prelude::*, render::{ - extract_component::{ExtractComponentPlugin, UniformComponentPlugin}, render_graph::{RenderGraphApp, ViewNodeRunner}, RenderApp + extract_component::{ExtractComponentPlugin, UniformComponentPlugin}, + render_graph::{RenderGraphApp, ViewNodeRunner}, + RenderApp, }, }; @@ -31,7 +33,8 @@ impl Plugin for OutlinePostProcessPlugin { app.add_plugins(( UniformComponentPlugin::<components::OutlinePostProcessSettings>::default(), ExtractComponentPlugin::<components::OutlinePostProcessSettings>::default(), - )).add_systems(Update, update_shader_clip_planes); + )) + .add_systems(Update, update_shader_clip_planes); let Some(render_app) = app.get_sub_app_mut(RenderApp) else { return; |